JavaScript Class # OOPS JS
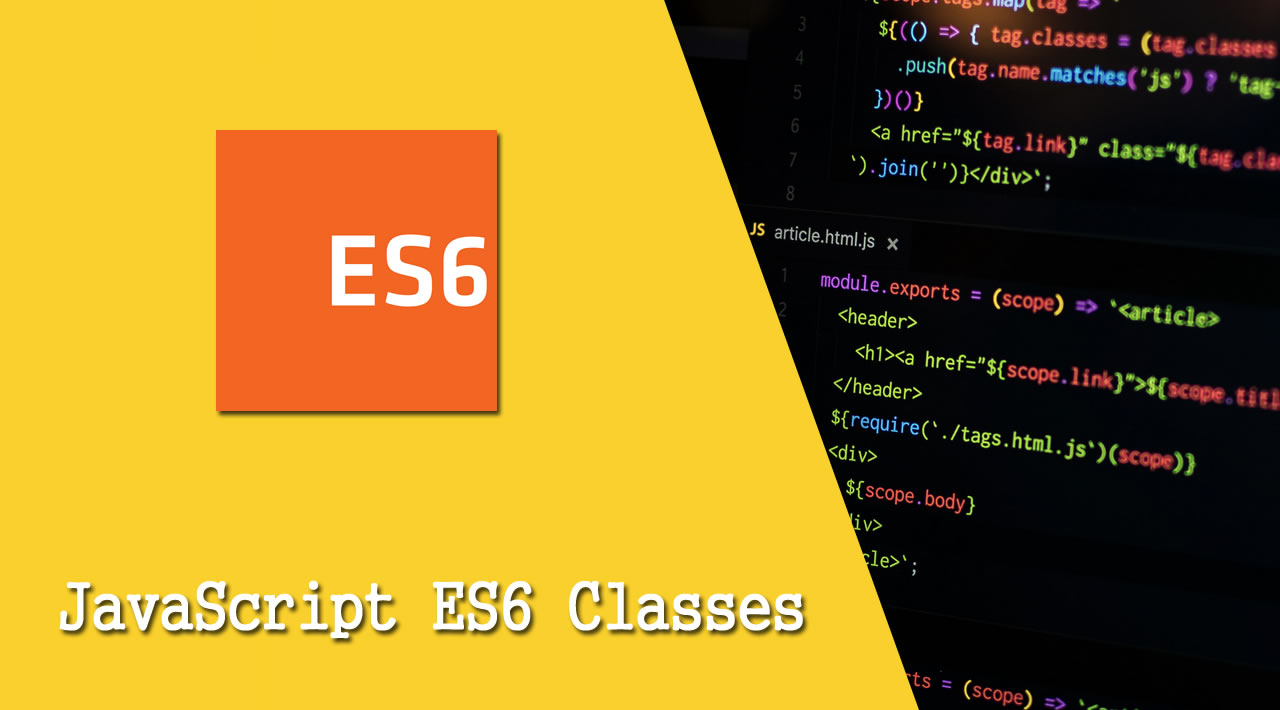
JavaScript ES6 Classes
An exciting new construct that was introduced in the ES6 specification is the ES6 classes. If you're a Javascript developer, you will be aware that Javascript follows prototypal inheritance and sometimes it can get a little messy. However, with ES6 classes the syntax is simpler and much more intuitive.
Classes are a fundamental part of object oriented programming (OOP). They define “blueprints” for real-world object modeling and organize code into logical, reusable parts. Classes share many similarities with regular objects. They have their own constructor functions, properties, and methods. In this tutorial, we’ll demonstrate how to create and work with ES6 classes.
Creating a class
You can create a class using the class keyword:
class Patient{
constructor(name, age) {
this.name = name
this.age = age
}
}
let pat = new Patient("RK", 34)
pat.name
//returns 'RK'
pat.age
//returns 34
Notice how we define our Person class with a constructor() function taking two arguments name and age. Using the this keyword, we set the name and age properties based on the provided arguments. Remember that the constructor function is called whenever we create a new instance of the Person class.
Similar to objects, we can read class properties using dot notation so that person.name returns Sam.
Defining properties and methods
You can define properties and methods for a class the same way you do for regular objects:
class Patient{
constructor(name, age) {
this.name = name
this.age = age
}
sayPatientName() {
console.log("Patient name is " + this.name)
}
}
let patient = new Person('RK', 40)
patient.sayPatientName()
//logs 'Patient name is RK'
patient.location = 'Dindigul'
patient.location
//returns 'Dindigul'
Notice how we define a sayPatientName() function within our Person class definition. Once we create a new instance of Patient, we can call the method via patient.sayPatientName().
You can also add properties and methods on the fly. You’ll notice that while the location property isn’t defined in our constructor function, we can still dynamically add it later on for the person instance. Remember that if we created a new instance of Patient, it would not have a location property because that property is not defined in the class definition. Only properties and methods that we explicitly define will be shared by all instances of the class.
Static functions
You can use the static keyword to make class methods static. A static method acts on the class itself, not on instances of the class:
class Patient{
constructor(name, age) {
this.name = name
this.age = age
}
static describe(){
console.log("This is a patient.")
}
sayName() {
console.log("My name is " + this.name)
}
}
Patient.describe()
//logs 'This is a patient.'
Notice how static methods operate on the class itself and not an instance of the class. We didn’t have to create a new Patient to call the static method.
Static methods are useful for common or shared class functionality. In this case, the describe() method is used to describe what the Patient class is. It will apply to every instance of Patient. This is why we make it a static method.
Class Inheritance
Inheritance allows you to create new classes based off existing ones. These new classes “inherit” the methods and properties of their parent. They can also override or extend the parent:
class Patient{
constructor(name, age) {
this.name = name
this.age = age
}
static describe(){
console.log("This is a Patient.")
}
sayName() {
console.log("My name is " + this.name)
}
}
class Doctor extends Patient {
sayName(){
console.log("Patient name is " + this.name + " and I am a Doctor !")
}
}
let rk = new Patient('Ravi', 40)
let doctor = new Doctor('Nethra', 33)
rk.sayName()
//logs 'My name is Ravi'
doctor.sayName()
//logs 'Patient name is Ravi and I am a Doctor !'
Using the extends keyword, we can create a new class sharing the same characteristics as Patient. Notice how we override the sayName() method with a new definition for the Programmer class. Apart from overriding this method, everything else remains the same for both Patient and Doctor.
Using super
The super keyword allows a child class to invoke parent class properties and methods.
class Patient{
constructor(name, age) {
this.name = name
this.age = age
}
static describe(){
console.log("This is a patient.")
}
sayName() {
console.log("Patient name is " + this.name)
}
}
class Doctor extends Patient {
sayName(){
super.sayName()
console.log("My name is " + this.name + " and I am a Doctor!")
}
}
let rk = new Person('Ravi', 40)
let doctor = new Doctor('Nethra', 33)
doctor.sayName()
//logs 'My name is Ravi'
//logs 'My name is Nethra and I am a Doctor !'
Notice how we call super.sayName() in the Doctor implementation of sayName(). While this invokes the parent implementation of super.sayName(), the name property still references the Programmer class.
Conclusion
Classes facilitate object oriented programming in JavaScript. While regular objects provide similar functionality, classes provide the extra advantage of inheritance and static methods.
Comments
Post a Comment